Overview of SQL Joins
SQL Joins are fundamental in relational databases to combine rows from two or more tables. The type of join used determines how rows from the joined tables are matched and what results are returned. Understanding the different joins is crucial for data manipulation and analysis.
- INNER JOIN: Returns rows when there is at least one match in both tables. If no match is found, rows are not returned.
- LEFT JOIN (LEFT OUTER JOIN): Returns all rows from the left table, and matched rows from the right table. If there is no match, the result from the right side will be NULL.
- RIGHT JOIN (RIGHT OUTER JOIN): Returns all rows from the right table, and matched rows from the left table. If there is no match, the result from the left side will be NULL.
- FULL JOIN (FULL OUTER JOIN): Combines the results of both LEFT JOIN and RIGHT JOIN. It returns all rows from both tables, with NULLs in place where there is no match.
- CROSS JOIN: Returns a Cartesian product of the two tables, i.e., all possible combinations of rows from the joined tables. No matching condition is used.
- SELF JOIN, NATURAL JOIN, EQUI JOIN, ANTI JOIN, and SEMI JOIN: These are variations or implementations using the basic join types to fulfill specific data querying needs, like comparing rows within the same table or filtering non-matching rows.
Each join type serves different data retrieval and analysis needs. Choosing the right join type is key to accurate data representation and efficient database queries. Let us learn in detail.
1. Introduction to SQL Joins
SQL Joins are used to combine rows from two or more tables, based on a related column between them. There are several types of joins: INNER JOIN, LEFT JOIN (LEFT OUTER JOIN), RIGHT JOIN (RIGHT OUTER JOIN), FULL JOIN (FULL OUTER JOIN), and CROSS JOIN. Each type of join serves a specific purpose and facilitates the retrieval of data from multiple tables in a relational database.
1.1 INNER JOIN
The INNER JOIN keyword selects records that have matching values in both tables. Only the rows with matching keys in both tables are returned.
SELECT table1.column1, table2.column2
FROM table1
INNER JOIN table2
ON table1.common_field = table2.common_field;
This query returns rows that have matching values in both tables involved in the join.
1.2 LEFT JOIN (LEFT OUTER JOIN)
The LEFT JOIN returns all records from the left table, and the matched records from the right table. The result is NULL from the right side if there is no match.
SELECT table1.column1, table2.column2
FROM table1
LEFT JOIN table2
ON table1.common_field = table2.common_field;
Even if there are no matches in the right table, all rows from the left table are included in the results.
1.3 RIGHT JOIN (RIGHT OUTER JOIN)
The RIGHT JOIN keyword returns all records from the right table, and the matched records from the left table. The result is NULL from the left side when there is no match.
SELECT table1.column1, table2.column2
FROM table1
RIGHT JOIN table2
ON table1.common_field = table2.common_field;
This join ensures that all rows from the right table appear in the joined table, with nulls in columns from the left table when there is no match.
1.4 FULL JOIN (FULL OUTER JOIN)
The FULL JOIN keyword returns all records when there is a match in either left (table1) or right (table2) table records. Records not matching across tables will have NULL values for every column of the table that lacks a matching row.
SELECT table1.column1, table2.column2
FROM table1
FULL JOIN table2
ON table1.common_field = table2.common_field;
This join combines the results of both LEFT JOIN and RIGHT JOIN.
1.5 CROSS JOIN
The CROSS JOIN keyword produces a Cartesian product of the two tables involved in the join. This means it returns a combined set that contains all possible combinations of rows from the joined tables.
SELECT table1.column1, table2.column2
FROM table1
CROSS JOIN table2;
This type of join does not necessitate a joining condition. The result set is the number of rows in the first table multiplied by the number of rows in the second table.
1.5.1 Practical Uses of CROSS JOIN
CROSS JOIN can be used to generate exhaustive combinations of records from two tables which can be useful for creating combinations of products, for testing, or for generating reports that require every permutation of a given set of data.
1.6 Combining Joins
SQL allows for the combination of different types of joins in a single query. This capability is powerful for constructing complex queries that require data from multiple tables to be combined in various ways. Careful construction of the SQL statement ensures that data integrity and query performance are maintained.
SELECT table1.column1, table2.column2, table3.column3
FROM table1
INNER JOIN table2 ON table1.common_field = table2.common_field
LEFT JOIN table3 ON table1.common_field = table3.common_field;
This example shows an INNER JOIN and a LEFT JOIN being used together to join data from three tables.
1.6.1 Considerations for Combining Joins
When combining joins, it's important to consider the order of operations and the logic of how tables are connected. Incorrect join conditions can lead to unexpected results or performance issues. Planning and testing join conditions carefully ensures accurate and efficient queries.
2. Understanding INNER JOIN
The INNER JOIN keyword in SQL is used to combine rows from two or more tables, based on a related column between them. This type of join returns rows only when there is at least one match in both tables. If there are rows in one table that do not have matches in the other, those rows will not be included in the result set.
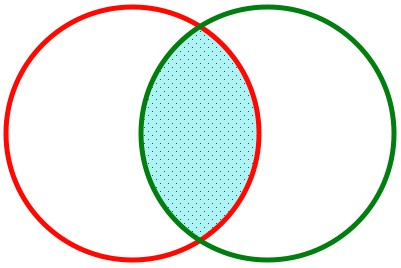
2.1 Syntax of INNER JOIN
The basic syntax for an INNER JOIN is as follows:
SELECT columns
FROM table1
INNER JOIN table2
ON table1.common_column = table2.common_column;
This query selects columns from two tables that are joined based on a condition that matches columns from both tables.
2.2 Example of INNER JOIN
Consider two tables, Employees and Departments, where each employee is assigned to a department. An example query to find the department for each employee would be:
SELECT Employees.Name, Departments.DepartmentName
FROM Employees
INNER JOIN Departments ON Employees.DepartmentID = Departments.ID;
This query will return the names of employees along with the names of their respective departments, excluding any employees without a department.
2.3 Joining Multiple Tables
INNER JOIN can also be used to join more than two tables in a single query, allowing for complex data retrieval. For example, to add an Offices table that maps employees to their office locations:
SELECT Employees.Name, Departments.DepartmentName, Offices.Location
FROM Employees
INNER JOIN Departments ON Employees.DepartmentID = Departments.ID
INNER JOIN Offices ON Employees.OfficeID = Offices.ID;
This query retrieves not only the department name for each employee but also their office location, demonstrating how INNER JOIN can be cascaded to include multiple tables.
2.3.1 Using Aliases with INNER JOIN
Aliases can simplify queries, especially with multiple joins, by providing a shorthand for table names. For example:
SELECT e.Name, d.DepartmentName, o.Location
FROM Employees e
INNER JOIN Departments d ON e.DepartmentID = d.ID
INNER JOIN Offices o ON e.OfficeID = o.ID;
Here, aliases 'e' for Employees, 'd' for Departments, and 'o' for Offices make the query more readable.
2.4 Filtering Results with INNER JOIN
Using the WHERE clause with INNER JOIN allows for filtering the results based on specific criteria. For instance, to find employees in a specific department:
SELECT e.Name, d.DepartmentName
FROM Employees e
INNER JOIN Departments d ON e.DepartmentID = d.ID
WHERE d.DepartmentName = 'Engineering';
This query will return only the names of employees who are in the Engineering department.
2.5 Aggregation and INNER JOIN
INNER JOIN can be used in conjunction with aggregation functions to perform operations like counting, averaging, or summing values from joined tables. For example, to count the number of employees in each department:
SELECT d.DepartmentName, COUNT(e.ID) AS NumberOfEmployees
FROM Departments d
INNER JOIN Employees e ON d.ID = e.DepartmentID
GROUP BY d.DepartmentName;
This query demonstrates how to combine INNER JOIN with GROUP BY and COUNT to aggregate data across joined tables.
3. Understanding Left Join
A Left Join in SQL, also known as a Left Outer Join, is a method for combining rows from two or more tables based on a related column between them. The Left Join returns all records from the left table, and the matched records from the right table. If there is no match, the result is NULL on the side of the right table.
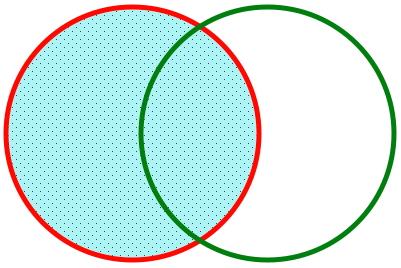
3.1 Syntax and Basic Example
The basic syntax for a Left Join in SQL is as follows:
SELECT columns
FROM table1
LEFT JOIN table2
ON table1.common_field = table2.common_field;
An example to demonstrate the Left Join:
SELECT Employees.Name, Departments.Name
FROM Employees
LEFT JOIN Departments ON Employees.DeptID = Departments.ID;
This query retrieves all employees and their department names. Employees not associated with a department will have NULL in the department name column.
3.2 Conditions and Filtering
Left Join can also be combined with WHERE clause to filter the results based on certain conditions.
SELECT Employees.Name, Departments.Name
FROM Employees
LEFT JOIN Departments ON Employees.DeptID = Departments.ID
WHERE Departments.Name IS NOT NULL;
This query shows only those employees who belong to a department, effectively filtering out rows where the department name would be NULL.
3.3 Using Left Join to Solve Problems
Left Join is particularly useful in scenarios where you need to find records in one table that have no corresponding record in another table.
SELECT Employees.Name
FROM Employees
LEFT JOIN Departments ON Employees.DeptID = Departments.ID
WHERE Departments.ID IS NULL;
This query finds employees who are not assigned to any department.
3.3.1 Performance Considerations
While Left Joins are powerful, they can impact database performance, especially with large datasets. Proper indexing on the join columns can significantly improve query performance. Additionally, it's important to only select the columns that are necessary for your query to minimize the amount of data processed and transferred.
3.4 Complex Joins
Left Joins can be nested or combined with other types of joins to solve more complex queries. For example, combining Left Join with Inner Join:
SELECT Employees.Name, Departments.Name, Offices.Location
FROM Employees
LEFT JOIN Departments ON Employees.DeptID = Departments.ID
INNER JOIN Offices ON Employees.OfficeID = Offices.ID;
This query retrieves all employees, their department names, and office locations. If an employee does not belong to a department, the department name will be NULL, but every employee must have an office location due to the inner join with the Offices table.
3.4.1 Aggregates with Left Join
Left Join is also useful when working with aggregate functions to include all records from the left table in the aggregation:
SELECT Departments.Name, COUNT(Employees.ID) AS EmployeeCount
FROM Departments
LEFT JOIN Employees ON Departments.ID = Employees.DeptID
GROUP BY Departments.Name;
This query counts the number of employees in each department, including departments with no employees.
3.5 Self Join with Left Join
A Self Join is a join where a table is joined with itself. When combined with Left Join, it can be used to compare records within the same table.
SELECT e1.Name AS Employee1, e2.Name AS Employee2
FROM Employees e1
LEFT JOIN Employees e2 ON e1.ManagerID = e2.ID;
This query retrieves pairs of employees where one is the manager of the other. Employees without a manager will have NULL in the Employee2 column.
3.5.1 Recursive Queries
Self Joins with Left Join are also used in recursive queries where a table has a hierarchical relationship with itself, such as an employee reporting structure or a product category hierarchy.
3.6 LEFT JOIN with NULL Checks
Using a LEFT JOIN in SQL allows you to combine rows from two tables based on a related column, returning all rows from the left table and the matched rows from the right table, with NULLs for non-matching rows from the right table. Incorporating NULL checks in your query enables you to filter results based on the presence or absence of matches.
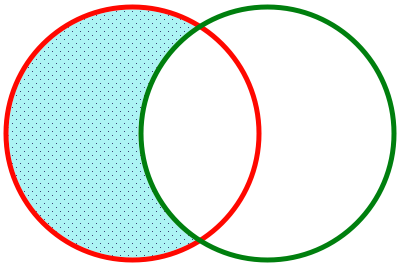
Basic Syntax
SELECT table1.column, table2.column
FROM table1
LEFT JOIN table2 ON table1.common_column = table2.common_column
WHERE table2.common_column IS NULL;
This syntax is used to find rows in the left table that have no corresponding rows in the right table.
Use Case: Identifying Unmatched Rows
To identify rows in table1
that do not have a match in table2
, the WHERE clause checks for NULL values in a column of table2
that would be NULL due to the LEFT JOIN.
Example Query
SELECT Employees.Name
FROM Employees
LEFT JOIN Departments ON Employees.DepartmentID = Departments.ID
WHERE Departments.ID IS NULL;
This query finds employees who are not assigned to any department. The WHERE Departments.ID IS NULL
clause filters out all employees that do have a department, leaving only those without one.
Considerations
- NULL checks are crucial for filtering results after a LEFT JOIN to either include or exclude rows based on the existence of matches.
- Indexing the columns used in the JOIN condition can improve performance, especially for large datasets.
- Understanding how NULL values are handled in SQL is essential for accurately interpreting the results of queries with LEFT JOINs and NULL checks.
4. Understanding RIGHT JOIN
RIGHT JOIN, also known as RIGHT OUTER JOIN, is a type of SQL join that returns all rows from the right table and the matched rows from the left table. If there is no match, the result will include NULL values for columns from the left table. This join is especially useful for finding records in the right table that have no corresponding records in the left table.
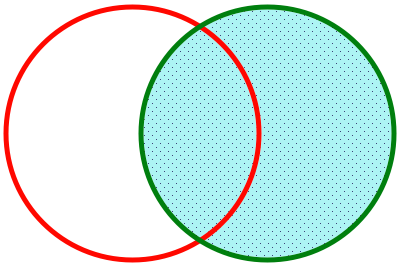
4.1 Syntax of RIGHT JOIN
The syntax for a RIGHT JOIN is as follows:
SELECT columns
FROM table1
RIGHT JOIN table2
ON table1.common_column = table2.common_column;
This query will fetch the specified columns from both tables where the join condition is met, including all rows from the right table even if there are no corresponding matches in the left table.
4.2 Example of RIGHT JOIN
Consider a database with two tables: Orders and Customers. To find all customers and any orders they might have, you could use the following query:
SELECT Customers.CustomerName, Orders.OrderID
FROM Orders
RIGHT JOIN Customers ON Orders.CustomerID = Customers.CustomerID;
This query returns all customers, including those who haven't placed any orders, with NULL values for OrderID where there are no matching orders.
4.3 Differences Between LEFT JOIN and RIGHT JOIN
While LEFT JOIN and RIGHT JOIN are similar in their functionality, the main difference lies in the direction of the join. LEFT JOIN fetches all rows from the left table and matched rows from the right table, whereas RIGHT JOIN does the opposite, focusing on the right table as the primary source of data.
In practice, you can often achieve the same results with either type of join by switching the order of the tables in the FROM and JOIN clauses.
4.4 Using RIGHT JOIN for Data Analysis
RIGHT JOIN is particularly useful in data analysis for identifying discrepancies or missing data. For example, in a database tracking inventory and sales, a RIGHT JOIN could identify products that have never been sold:
SELECT Products.ProductName, Sales.SaleID
FROM Sales
RIGHT JOIN Products ON Sales.ProductID = Products.ProductID
WHERE Sales.SaleID IS NULL;
This query highlights products in the inventory that haven't been sold, by looking for NULL values in the SaleID column resulting from the RIGHT JOIN.
4.4.1 Combining RIGHT JOIN with WHERE Clause
Filtering results with the WHERE clause after performing a RIGHT JOIN can refine the query outputs to meet specific criteria:
SELECT Customers.CustomerName, Orders.OrderID
FROM Orders
RIGHT JOIN Customers ON Orders.CustomerID = Customers.CustomerID
WHERE Orders.OrderDate >= '2023-01-01';
This query would return all customers and any orders placed on or after January 1, 2023. Customers without orders in this period would still be listed, with NULL values for their OrderID.
4.5 RIGHT JOIN and NULL Values
Understanding how RIGHT JOIN handles NULL values is crucial. When the join condition fails (i.e., there is no matching row in the left table for a row in the right table), the query result will include the row from the right table combined with NULL values for each column from the left table. This behavior is instrumental in identifying unmatched records in the primary (right) table.
4.6 Practical Tips for Using RIGHT JOIN
- Use RIGHT JOIN when you are more interested in the rows from the right table.
- Remember to check for NULL values in your WHERE clause to include or exclude unmatched rows effectively.
- Consider using LEFT JOIN instead if it makes the query more intuitive, as RIGHT JOIN can sometimes be less commonly used and harder to read for some people.
4.7 RIGHT JOIN with NULL Checks
A RIGHT JOIN, or RIGHT OUTER JOIN, in SQL, pairs all rows from the right table with matching rows from the left table. Rows in the right table that do not find a match in the left table are still included in the result set, with NULL values for the columns of the left table. NULL checks within a RIGHT JOIN query allow for specific filtering based on the presence or absence of matching data.
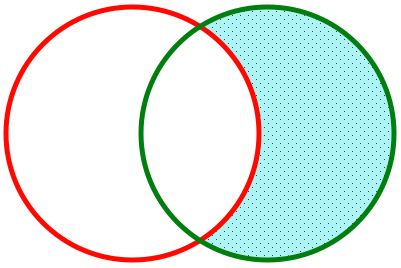
Basic Syntax
SELECT table1.column, table2.column
FROM table1
RIGHT JOIN table2 ON table1.common_column = table2.common_column
WHERE table1.common_column IS NULL;
This pattern is typically used to find rows in the right table that have no corresponding rows in the left table.
Use Case: Filtering for Unmatched Rows
To identify rows in table2
that lack a corresponding row in table1
, apply a WHERE clause that checks for NULL values in the joined column from table1
. This method effectively filters the result set to include only those rows from the right table without a match.
Example Query
SELECT Projects.ProjectName
FROM Employees
RIGHT JOIN Projects ON Employees.ProjectID = Projects.ID
WHERE Employees.ProjectID IS NULL;
This query lists all projects that currently have no employees assigned to them. By checking for NULL in Employees.ProjectID
, it filters out projects that do have employee assignments, highlighting those without.
Considerations
- Using NULL checks with RIGHT JOIN is instrumental in data analysis, especially when identifying missing associations or unfulfilled conditions between tables.
- Performance can be optimized by ensuring the columns involved in the join condition are indexed, thus speeding up the search for matches and non-matches.
- Understanding SQL's handling of NULL values is key to interpreting the results of RIGHT JOIN queries accurately, especially when combined with NULL checks.
5. Understanding FULL JOIN
FULL JOIN, also known as FULL OUTER JOIN, combines the results of both LEFT JOIN and RIGHT JOIN. It returns all rows from both tables, filling in with NULL values on the side that does not have a match. This type of join is useful for finding all records in two tables regardless of whether there is a match between them.
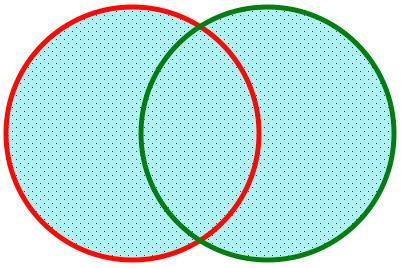
5.1 Syntax of FULL JOIN
The syntax for a FULL JOIN is as follows:
SELECT columns
FROM table1
FULL JOIN table2
ON table1.common_column = table2.common_column;
This query selects the specified columns from both table1 and table2, including all matches and non-matches from both sides.
5.2 Example of FULL JOIN
Consider two tables, Orders and Customers. To find all orders and customers, including those without a match (i.e., orders without customers and customers without orders), you can use a FULL JOIN:
SELECT Customers.CustomerName, Orders.OrderID
FROM Customers
FULL JOIN Orders ON Customers.CustomerID = Orders.CustomerID;
This query returns all customers and orders, filling in with NULLs where there is no match (e.g., a customer who has not made any orders or an order with no associated customer).
5.3 Handling NULLs in FULL JOIN
In the result of a FULL JOIN, NULL values indicate the absence of a match. You can use the COALESCE function or similar to handle these NULL values, replacing them with a default value or another column's value for clearer results.
SELECT COALESCE(Customers.CustomerName, 'No Customer') AS CustomerName,
COALESCE(Orders.OrderID, 'No Order') AS OrderID
FROM Customers
FULL JOIN Orders ON Customers.CustomerID = Orders.CustomerID;
This modifies the output to replace NULLs with more informative placeholders, making the data easier to understand.
5.4 Benefits of FULL JOIN
FULL JOIN is especially beneficial when you need a complete overview of two tables' relationships, including all matches and non-matches. It is ideal for comprehensive comparisons, data auditing, and identifying discrepancies between tables.
5.4.1 Using FULL JOIN for Data Reconciliation
FULL JOIN is particularly useful for data reconciliation tasks, allowing you to compare records in two tables to find matches, discrepancies, and missing entries in either table. This capability is crucial for data integrity checks and ensuring consistency across related datasets.
5.5 Limitations and Considerations
While FULL JOIN is powerful, it can produce large result sets, especially with tables that have many non-matching rows. This can impact performance and readability. It's important to use FULL JOIN judiciously and consider filtering the results with WHERE clauses to focus on specific areas of interest.
5.6 Practical Examples and Uses
FULL JOIN is often used in reporting and data analysis to provide a comprehensive view of related data from different sources. For example, in a sales database, a FULL JOIN can be used to identify all products and sales transactions, highlighting unsold products and customers who haven't made purchases.
5.7 FULL JOIN with NULL Checks
A FULL JOIN, or FULL OUTER JOIN, combines the results of both LEFT JOIN and RIGHT JOIN. It returns all rows from both tables, with NULLs in places where there is no match. Implementing NULL checks in a FULL JOIN query allows you to identify rows that only appear in one of the two tables or to filter out such rows based on your requirements.
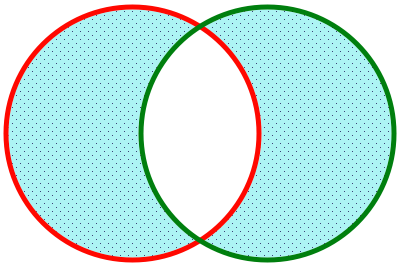
Basic Syntax
SELECT table1.column, table2.column
FROM table1
FULL JOIN table2 ON table1.common_column = table2.common_column
WHERE table1.common_column IS NULL OR table2.common_column IS NULL;
This syntax helps in identifying rows that are exclusive to either table by checking for NULLs in the joining columns after the FULL JOIN.
Use Case: Identifying Exclusive Rows
To find rows in table1
that have no corresponding rows in table2
and vice versa, the WHERE clause is used to check for NULL values in the columns of both tables.
Example Query
SELECT Employees.Name, Departments.Name
FROM Employees
FULL JOIN Departments ON Employees.DepartmentID = Departments.ID
WHERE Employees.DepartmentID IS NULL OR Departments.ID IS NULL;
This query identifies employees without a department and departments without any employees. It uses NULL checks to filter for rows where the join condition fails on either side.
Considerations
- Using FULL JOIN with NULL checks can significantly aid in data reconciliation tasks, helping to identify missing or unmatched records between two datasets.
- Be mindful of the potential for large result sets with FULL JOIN, especially in databases with large tables, as every combination of non-matching rows will be included.
- Ensure proper indexing on the columns used in the join condition to optimize query performance.
6. Understanding CROSS JOIN
CROSS JOIN, sometimes referred to as a Cartesian Join, produces a Cartesian product of the two tables involved in the join. This means it returns all possible combinations of rows from the first table with rows from the second table. Unlike other joins, CROSS JOIN does not require a joining condition to match rows from the joined tables.
6.1 Syntax of CROSS JOIN
The syntax for a CROSS JOIN is straightforward:
SELECT columns
FROM table1
CROSS JOIN table2;
This query selects columns from both table1 and table2, combining every row of table1 with every row of table2.
6.2 Example of CROSS JOIN
Consider two tables, Colors and Products. To find all possible combinations of colors and products, you can use a CROSS JOIN:
SELECT Colors.Color, Products.Product
FROM Colors
CROSS JOIN Products;
This query returns a result set where each product is matched with every color, useful for scenarios where you need to consider all potential combinations.
6.3 Uses of CROSS JOIN
CROSS JOIN can be especially useful in scenarios requiring exhaustive pairing of rows from two tables. This includes generating reports that require every permutation of certain data, such as product options, testing scenarios, or planning and scheduling tasks.
6.4 Limitations and Considerations
While CROSS JOIN can be a powerful tool, it is important to use it judiciously. The resulting dataset can be very large if both tables have a significant number of rows, potentially leading to performance issues. It's recommended to use CROSS JOIN only when a complete set of combinations is absolutely necessary.
6.4.1 Optimizing CROSS JOIN Queries
To manage the potentially large result sets produced by CROSS JOIN, consider filtering the rows of the input tables before the join operation. This can be achieved by using subqueries or temporary tables to limit the rows involved in the CROSS JOIN, thus improving performance and making the results more manageable.
6.5 Practical Example: Scenario Planning
A practical application of CROSS JOIN is in scenario planning, where you might need to consider all possible outcomes. For instance, if you're testing software across different operating systems and hardware configurations, a CROSS JOIN can help list all possible combinations to ensure comprehensive test coverage.
6.6 Comparison with Other Joins
Unlike INNER JOIN, LEFT JOIN, RIGHT JOIN, and FULL JOIN, the CROSS JOIN does not use a condition to match rows. This fundamental difference makes CROSS JOIN unique in its ability to combine every row from one table with every row from another, without considering matches or relationships between the tables.